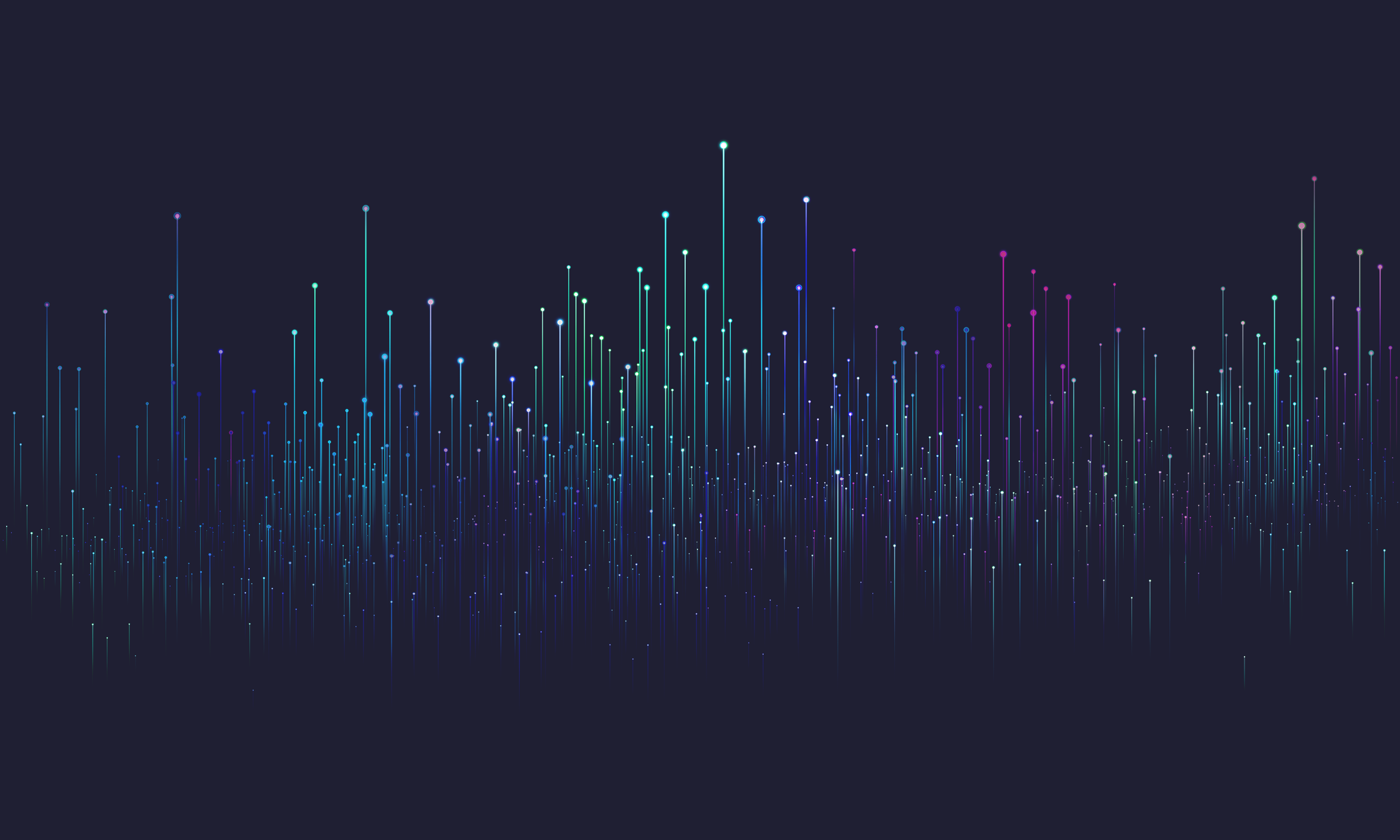
Entity API design
The article explains basic concepts of evitaDB entities, session handling, immutability and versioning mechanism. It also contains examples of working with the entity API from Java code.
All model classes are described by interfaces and there should not be any reason for using direct classes or instantiating them directly. The interfaces follow this structure:
- ModelNameContract - contains all read methods, represents the base contract for the model
- ModelNameEditor - contains all modification methods
- ModelNameBuilder - combines Contract + Editor, and it is actually used for building the instance
Versioning
This version information is used for two purposes:
- fast hashing + equality check: only the primaryKey + version information suffices to tell whether two instances are equal, and we can tell that with enough confidence even if only a part of the entity was really loaded from the persistent storage (if you need thorough comparison that compares all model data, you need to take advantage of the differsFrom method in the interface)
- optimistic locking: when there is a concurrent update of the same entity, we could automatically resolve the conflict, provided that the changes themselves do not overlap
This information may prove useful when this database goes into to distributed mode.
Removal
This decision has two roots:
- it's good to have the last version of the data when things go wrong, because we can still restore the previous value
- it's easy implementation-wise, and it doesn't require data deletion
This decision quite complicates the work with the model data which is planned as follows:
- application loads entity from the database
- application deletes some data -> data stays in the entity but are marked as dropped
- application stores entity to the database, data is marked as dropped
- application loads entity again -> data doesn't contain any deleted items even if they are actually in DB, evitaDB will clean dropped data before handing the entity to the application
- application may create new data with the same "primary key" as previously removed data and store them back to the DB
- EvitaDB will overwrite previously dropped data with new ones - versioning continues from the dropped version (i.e. it doesn't start from one)
Working with entities from code
Usually the entity creation will look like this:
And then followed by an operation, which will create a new entity:
This way, the created entity can be immediately checked against the schema. This form of code is a condensed version, and it may be split into several parts, which will reveal the "builder" used in the process.
Creating entities in detached mode
Entities can be also created even when an EvitaDB instance is not at hand:
Session
Read only vs. Read-Write sessions
In the future, the read only sessions may be spread out to multiple read nodes, while the read-write sessions will need to communicate with the master node.